1. Introduction to Secure Coding Practices
In today’s digital world, where technology plays a pivotal role in both personal and professional aspects, security has become a fundamental component of software development. Secure coding practices refer to techniques and methodologies that ensure the code you write is secure from the ground up, reducing the likelihood of vulnerabilities. These practices are critical in defending against cyberattacks and data breaches, which can compromise sensitive information, disrupt services, and tarnish reputations.
Why Security in Software Development Is Important
Security isn’t just a concern for IT professionals or large corporations—it’s crucial for every developer. When you build software, whether it’s a website, mobile app, or cloud-based solution, there’s always the risk of it becoming a target for hackers. Implementing secure coding practices early on in the development process helps you avoid the time-consuming and costly process of fixing security issues after they’ve been exploited.
Hackers are becoming increasingly sophisticated, constantly searching for vulnerabilities to exploit. A single breach can expose thousands or even millions of records, leading to legal issues, loss of trust, and financial penalties. With data privacy laws like GDPR (General Data Protection Regulation) and CCPA (California Consumer Privacy Act) in place, companies are now legally required to protect user data, making secure coding more important than ever.
Common Security Threats to Be Aware Of
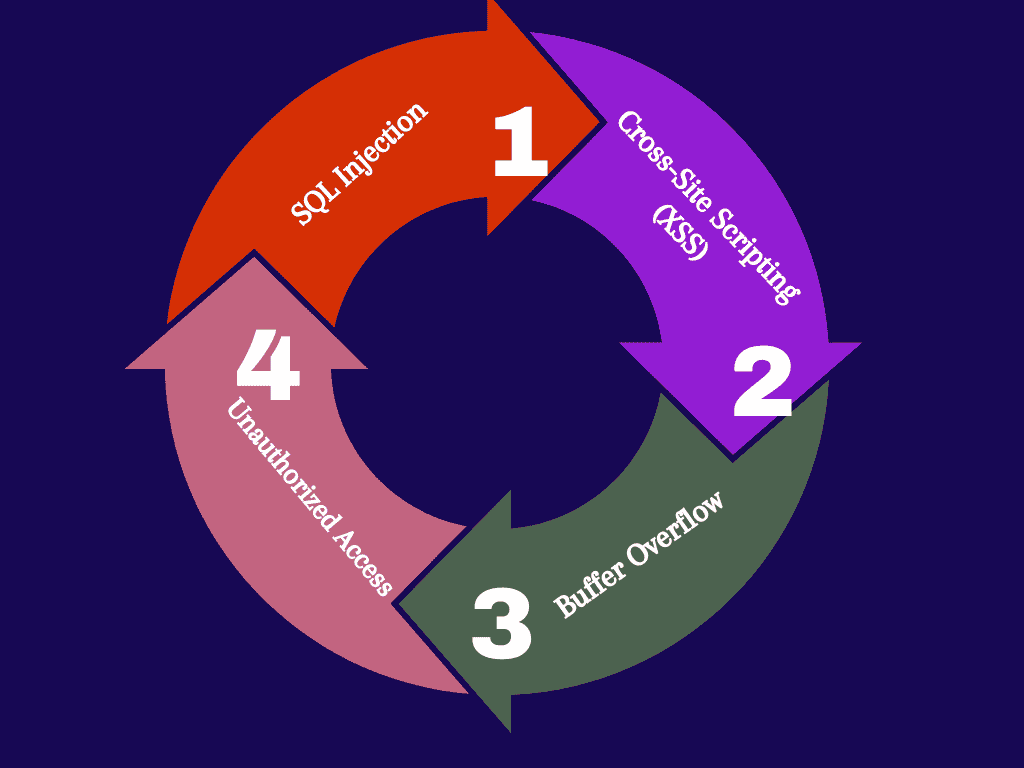
The first step in writing secure code is understanding the types of threats that exist. They are the more common.
- SQL Injection: A common attack where malicious SQL code is inserted into an entry field, allowing attackers to manipulate a database.
- Cross-Site Scripting (XSS): An attack where malicious scripts are injected into web pages viewed by others, potentially leading to data theft or session hijacking.
- Buffer Overflow: This occurs when a program writes more data to a buffer than it can hold, causing the program to crash or behave unpredictably.
- Unauthorized Access: This happens when an attacker gains access to areas of an application they are not supposed to, often due to weak authentication or authorization mechanisms.
Understanding these threats is critical in applying secure coding practices. By adopting a proactive approach to security, you can minimize the risk of vulnerabilities and protect your software from being exploited.
2. Secure Coding Practices for Input Validation
Input validation is one of the foundational secure coding practices. It’s all about ensuring that the data entering your system is exactly what you expect. If not handled properly, input data can become a gateway for attacks, particularly SQL injection and XSS attacks.
How to Prevent SQL Injection
“SQL injection is widely recognized as one of the most dangerous vulnerabilities in web security.”. It occurs when malicious users inject SQL queries into input fields, such as login forms or search bars, to manipulate your database. Once successful, an attacker can gain unauthorized access to data, modify records, or even delete entire databases.
Here are steps to prevent SQL injection:
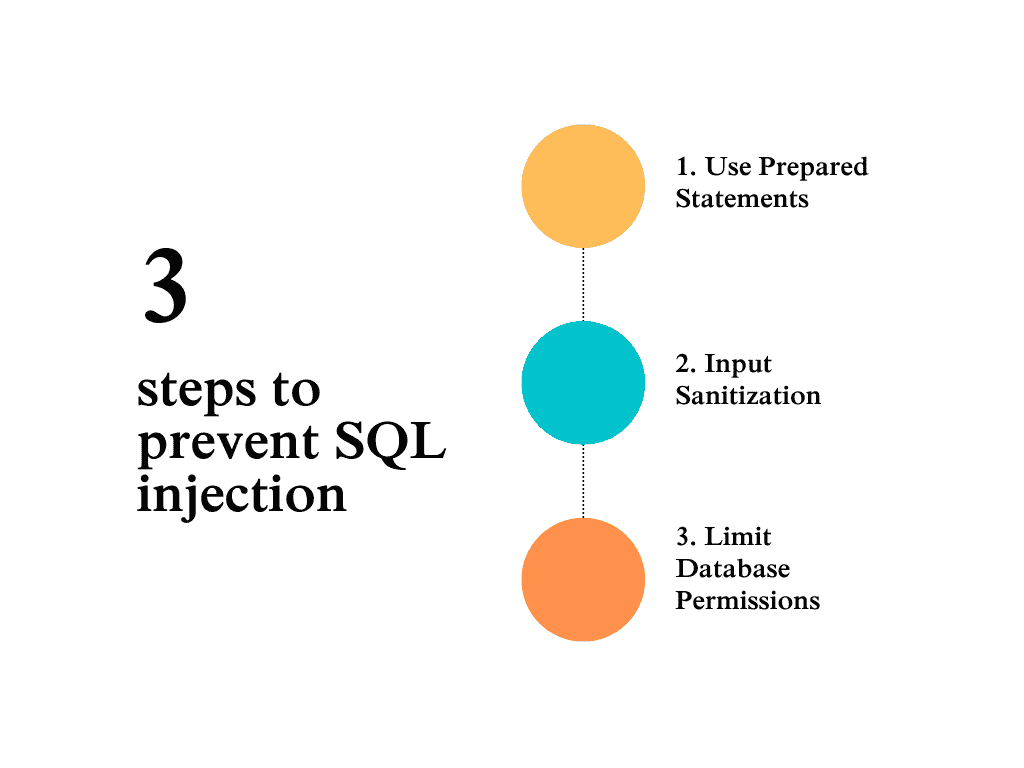
- Use Prepared Statements: Always use prepared statements with parameterized queries. Instead of directly inserting user input into your SQL queries, parameterized queries ensure that the input is treated as data, not executable code.Example (in PHP):phpCopy code
$stmt = $pdo->prepare("SELECT * FROM users WHERE username = ?"); $stmt->execute([$username]);
- Input Sanitization: Ensure that all user inputs are sanitized before being used in SQL queries. Remove or escape any special characters that could be used to manipulate the query.
- Limit Database Permissions: Use the principle of least privilege for your database users. For example, a web app should only have the permissions it needs to operate (e.g., SELECT, INSERT) and should not have full administrative access.
Stopping Cross-Site Scripting (XSS)
XSS attacks happen when malicious scripts are injected by attackers into web pages. These scripts can execute in the browsers of unsuspecting users, potentially stealing cookies, logging sessions, or performing actions on behalf of the user.
To mitigate XSS, consider the following:
Here are five ways to prevent Cross-Site Scripting (XSS), including the three you’ve mentioned:
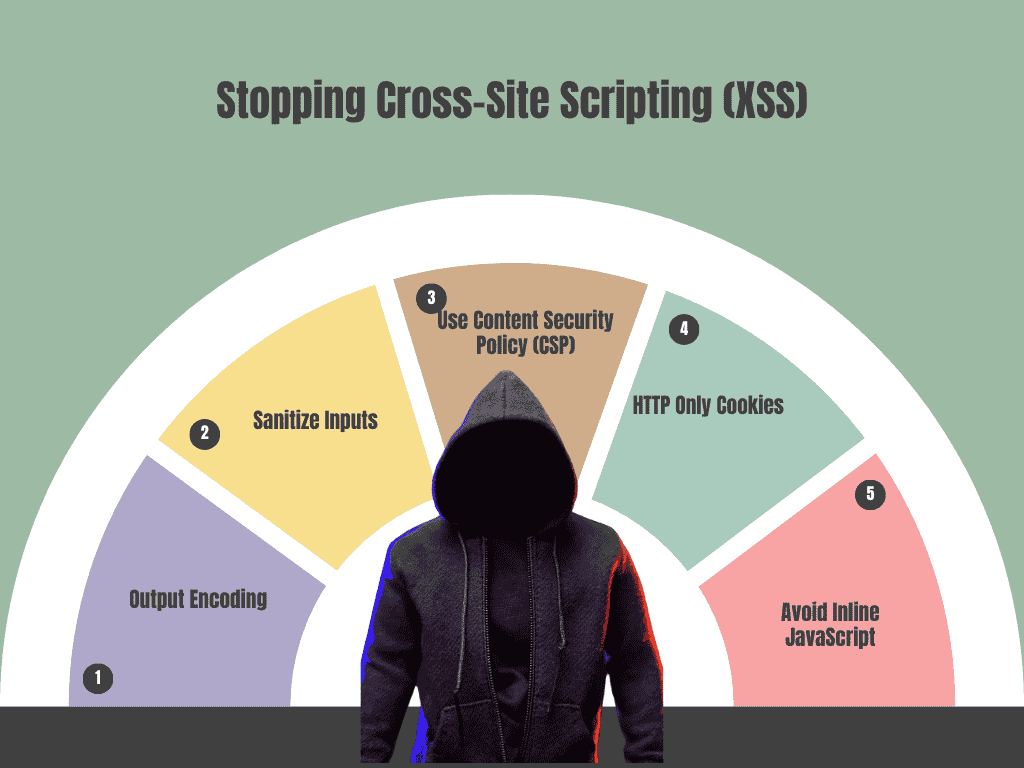
- Output Encoding: Encode user inputs before displaying them, converting characters like
<
and>
into their HTML entity equivalents (<
,>
), preventing them from being executed as code. - Sanitize Inputs: Always sanitize user inputs to remove any potentially malicious code before rendering it back to users, especially in form fields, URLs, or cookies.
- Use Content Security Policy (CSP): Implement CSP to limit the execution of scripts from only trusted sources, reducing the risk of XSS attacks.
- HTTP Only Cookies: Set the
HttpOnly
flag on cookies to prevent access to them through client-side scripts, reducing the risk of cookie theft during an XSS attack. - Avoid Inline JavaScript: Avoid placing JavaScript directly in HTML (inline), instead keeping scripts in separate files. This makes it easier to manage and apply CSP effectively.
Safely Handling User Inputs
In addition to the above, always assume that any user input could be malicious. This includes not only data from forms but also from cookies, query parameters, and HTTP headers. Implement a whitelist approach—allow only known safe inputs, rather than trying to filter out dangerous ones.
3. Secure Coding Practices for Authentication and Authorization
Authentication and authorization are core components of any secure application. These processes ensure that users are who they say they are and that they can only access the parts of your application they have permission to use.
Using Strong Authentication Mechanisms
A secure application starts with robust authentication mechanisms. This involves more than just usernames and passwords.
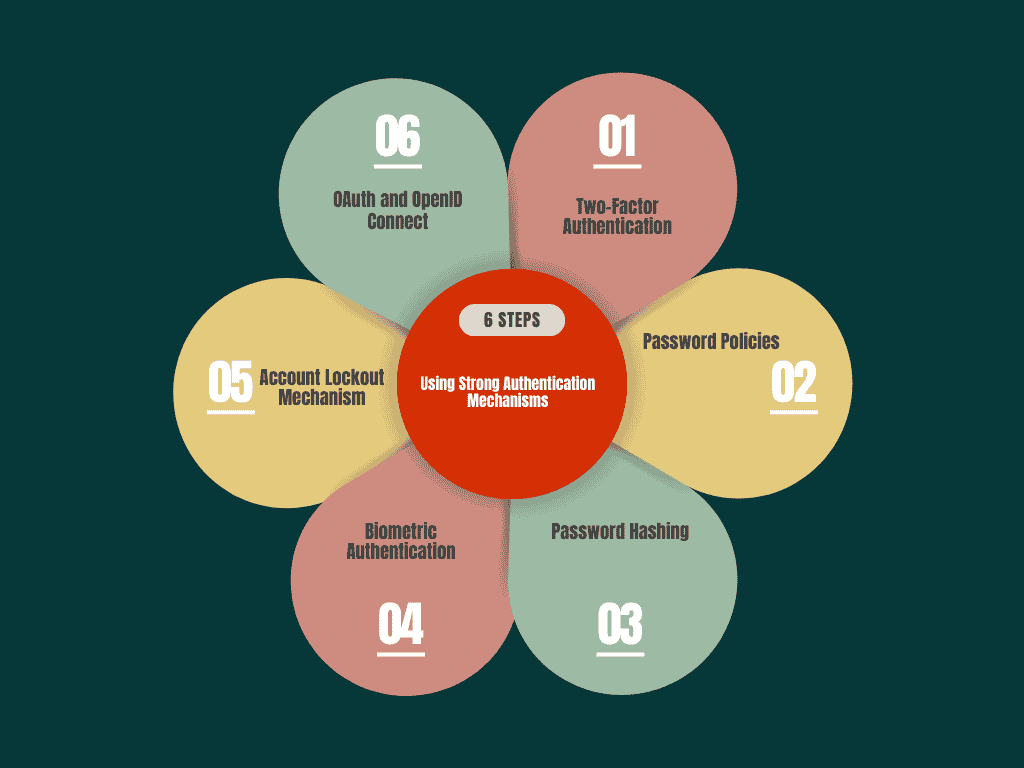
1Two-Factor Authentication (2FA): Add an extra layer of security by requiring a second factor, such as a text message code or an authentication app, along with the user’s password.
2 Password Policies: Enforce strong password requirements, such as using a mix of uppercase, lowercase, numbers, and symbols, and mandate regular password changes to reduce vulnerability.
3 Password Hashing: Use strong hashing algorithms like bcrypt or Argon2 to store passwords securely, ensuring that even if attackers gain access to your database, they cannot easily recover the passwords.
4 Biometric Authentication: Implement biometrics like fingerprint scanning, facial recognition, or iris scans, providing a more secure and convenient alternative to traditional passwords.
5 Account Lockout Mechanism: After a set number of failed login attempts, lock the account temporarily to prevent brute force attacks.
6 OAuth and OpenID Connect: Use federated identity protocols like OAuth and OpenID Connect to enable secure, token-based authentication, reducing the need for password management altogether.
Best Practices for Role-Based Access Control (RBAC)
RBAC is a method of managing user access based on their roles within an organization. By assigning users to roles and giving roles specific permissions, you can minimize the risk of unauthorized access.
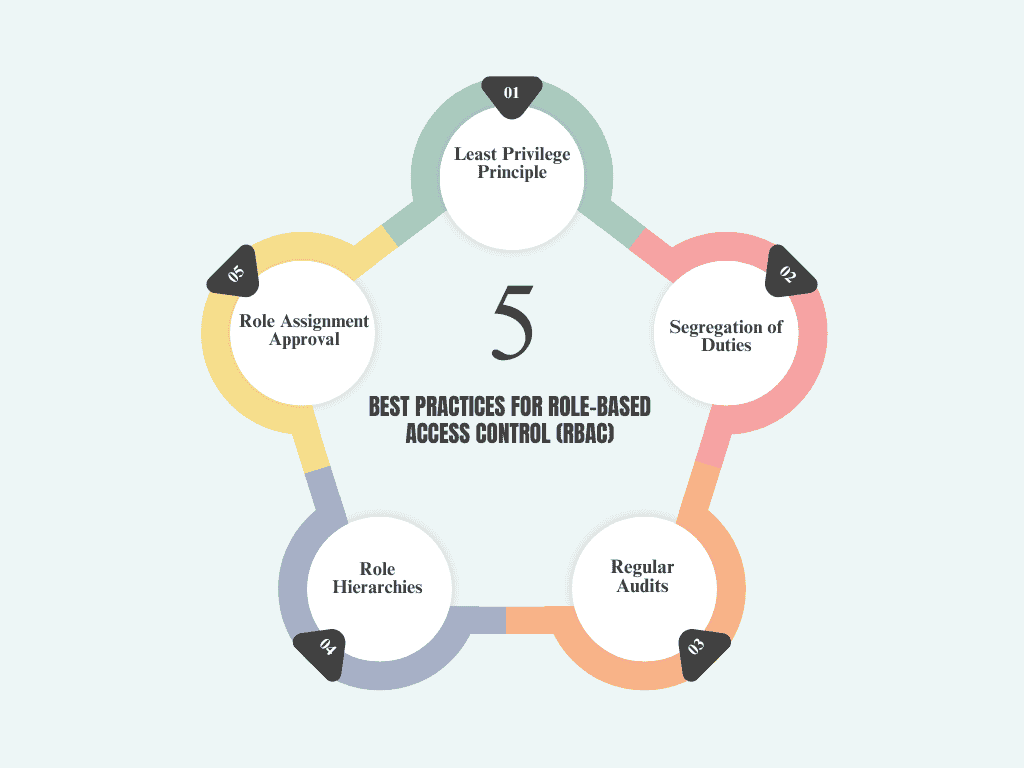
- Least Privilege Principle: Ensure users have only the minimal access required to perform their duties. Avoid granting excessive permissions to reduce the impact of compromised accounts.
- Segregation of Duties: Design roles so that no single user can perform all critical tasks alone, minimizing the potential for abuse or mistakes. For example, separating responsibilities for requesting, approving, and implementing changes.
- Regular Audits: Periodically review and update user roles and permissions to align with current job responsibilities. This helps prevent users from retaining unnecessary access when their role changes.
- Role Hierarchies: Use a role hierarchy to define relationships between roles, with higher-level roles inheriting permissions from lower-level ones. This simplifies management while keeping access control organized.
- Role Assignment Approval: Implement a formal process for assigning roles that includes managerial approval. This ensures that access is granted appropriately and helps prevent unauthorized or unnecessary role assignments.
Avoiding Authorization Mistakes
A common security flaw is broken access control, where users are able to access data or perform actions they should not be able to. This often happens due to insufficient checks on user permissions.
Token-Based Authentication: Use token-based systems like JSON Web Tokens (JWT) to manage user sessions. These tokens can be verified and validated with each request to ensure that the user is authorized to perform specific actions.
Enforce Authorization Checks: Authorization should be checked for every request. Don’t assume that just because a user is authenticated, they should have access to all parts of the system.
4. Secure Coding Practices for Data Encryption
Encryption is a crucial component of any secure coding strategy. By encrypting sensitive data, you ensure that even if data is intercepted or accessed by unauthorized individuals, it remains unreadable without the correct encryption key.
Encrypting Data in Transit and at Rest
Sensitive data needs protection both while it is being transmitted over networks (in transit) and when it is stored in databases or files (at rest).
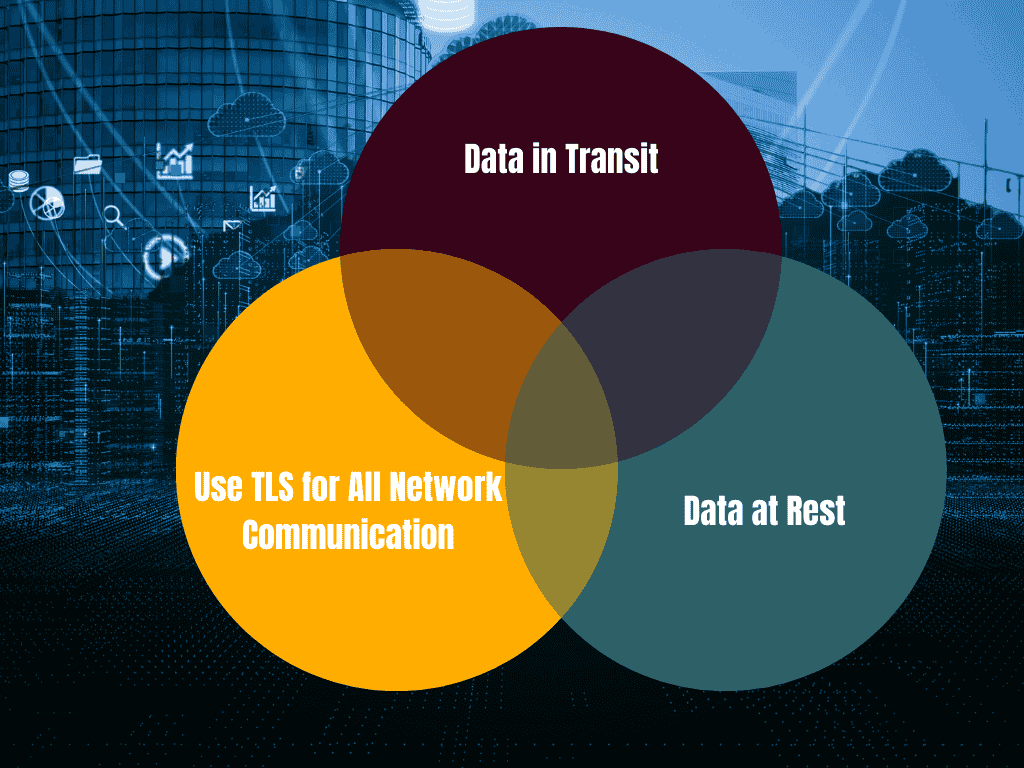
- Data in Transit: Always use secure communication channels such as HTTPS (Hypertext Transfer Protocol Secure) for web traffic. HTTPS encrypts data between the user’s browser and the server, preventing attackers from intercepting sensitive information such as login credentials or payment details.
- Data at Rest: Encryption should also be applied to data that is stored in databases or file systems. Sensitive information like personal identification numbers (PINs), credit card details, and health records should be encrypted using strong algorithms like AES-256 (Advanced Encryption Standard with a 256-bit key).
- Use TLS for All Network Communication: Transport Layer Security (TLS) is the standard protocol for securing network communications. By enforcing TLS, you can ensure that data exchanged between your application and external services is encrypted.
Choosing Strong Encryption Algorithms
The strength of your encryption relies heavily on the algorithms you use. Outdated encryption methods like DES (Data Encryption Standard) are no longer considered secure and should be avoided. Instead, opt for modern algorithms such as:
- AES (Advanced Encryption Standard): AES is the industry standard for encryption and is widely used for securing sensitive data. AES-256 is considered highly secure for most use cases.
- RSA (Rivest-Shamir-Adleman): RSA is commonly used for encrypting data exchanged between parties and for secure key exchange. It’s often used in conjunction with other encryption techniques.
It’s important to stay updated on encryption technologies, as what is secure today may not be secure tomorrow. For example, advancements in quantum computing could potentially break current encryption methods, making it essential to remain informed and ready to adopt new standards.
Managing Encryption Keys Securely
Encryption is only as secure as the method used to manage encryption keys. “Even the most robust encryption algorithms can be compromised by inadequate key management.
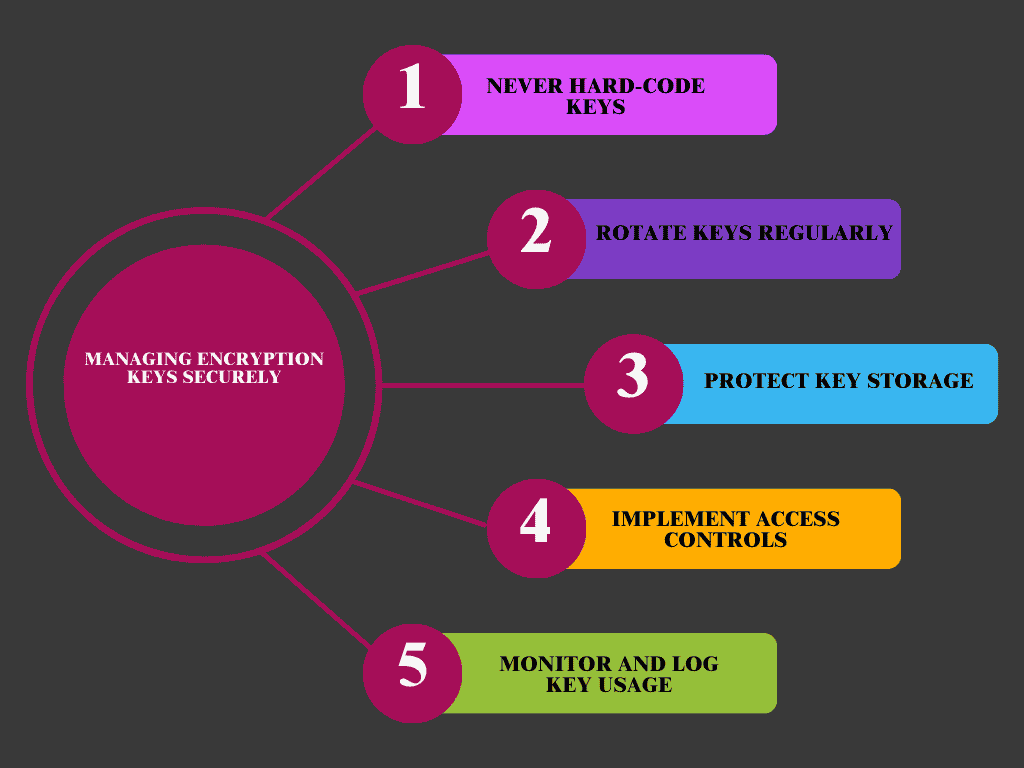
Here are five best practices for managing encryption keys securely, including the three you’ve provided:
- Never Hard-Code Keys: Avoid embedding encryption keys directly in your source code. Use secure key management services (e.g., AWS KMS, Google Cloud KMS) to keep keys separate and secure.
- Rotate Keys Regularly: Implement a schedule for regularly rotating encryption keys. This minimizes the risk of long-term exposure if a key is compromised, limiting its potential damage.
- Protect Key Storage: Store encryption keys separately from the data they protect, using secure hardware-based key management systems, such as Hardware Security Modules (HSMs).
- Implement Access Controls: Restrict access to encryption keys only to authorized personnel or systems. Use Role-Based Access Control (RBAC) to manage who can view or use the keys.
- Monitor and Log Key Usage: Implement logging and monitoring to track when and how encryption keys are used. This helps detect unauthorized access or potential misuse of the keys.
5. Secure Coding Practices for Error Handling
Error handling is an often-overlooked area of secure coding. Poor error handling can provide attackers with valuable insights into how your application operates, which can lead to the discovery of vulnerabilities.
Preventing Information Leaks in Error Messages
Error messages should never provide detailed information about the internal workings of your system, such as the structure of your database or the server’s configuration.
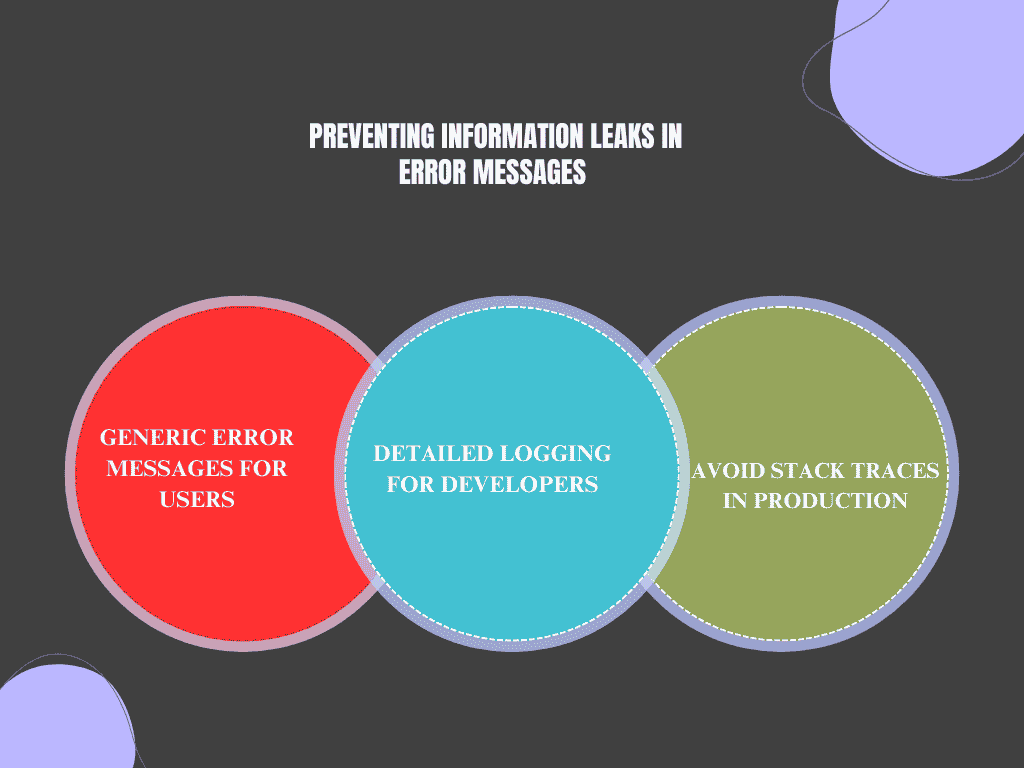
- Generic Error Messages for Users: When an error occurs, provide users with a generic message such as “An error has occurred. Please try again later.” Avoid giving specific details that could help an attacker understand your system’s weaknesses.
- Detailed Logging for Developers: While user-facing error messages should be vague, it’s essential to log detailed error information on the server side. These logs can help developers troubleshoot issues without exposing sensitive information to attackers.
- Avoid Stack Traces in Production: Displaying stack traces in production environments is a common mistake. Stack traces can give attackers insights into your codebase, including the programming languages, libraries, and frameworks you are using. Ensure that stack traces are disabled or hidden in production environments.
Logging and Monitoring Security Events
Strong logging and monitoring practices are crucial for identifying and addressing security incidents promptly. By tracking security-related events, you can identify patterns that may indicate an attempted attack.
- Log Security-Relevant Events: Make sure to log events such as failed login attempts, changes in user permissions, and access to sensitive data. This information can help you detect and respond to potential security incidents.
- Monitor Logs for Anomalies: Regularly monitor logs for suspicious activity. Tools such as intrusion detection systems (IDS) can automatically flag unusual patterns, such as repeated failed login attempts or unexpected changes in data.
- Ensure Log Integrity: It’s important to protect your logs from tampering. Store logs in a secure location, and consider using hashing techniques to verify their integrity. This ensures that if an attacker tries to modify the logs, the tampering will be detected.
Handling Exceptions Correctly
Proper exception handling ensures that your application can recover from unexpected errors without exposing sensitive information or crashing.
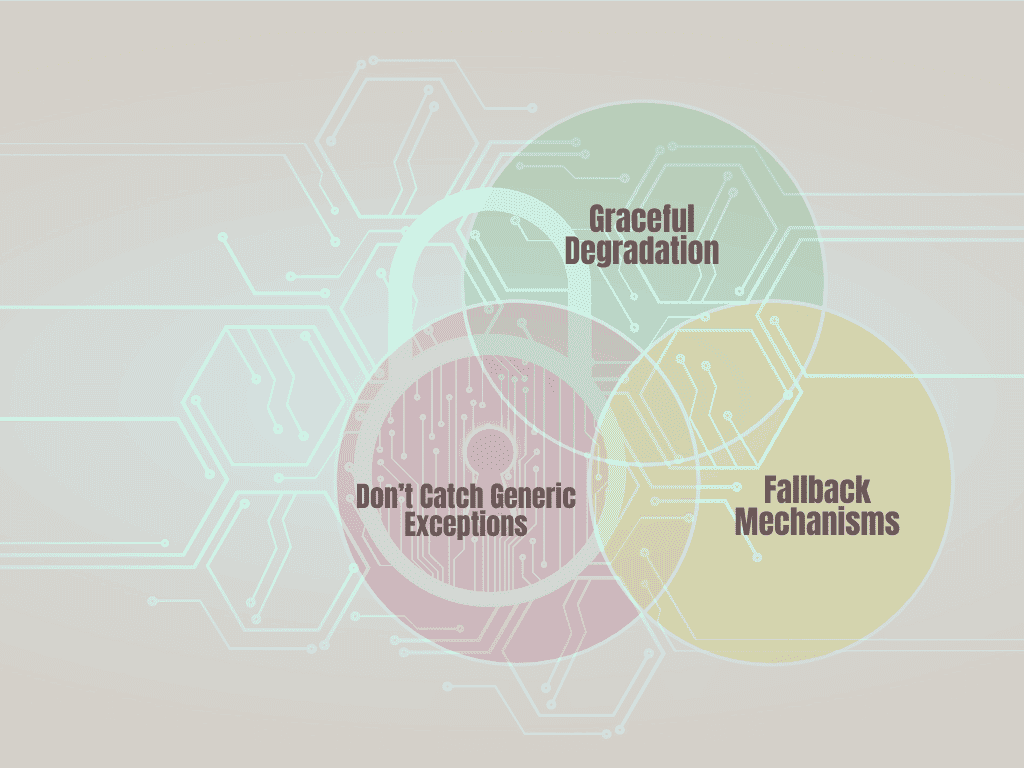
- Graceful Degradation: When an error occurs, your application should continue functioning, even if in a limited capacity. This is known as graceful degradation. For example, if a payment gateway is unavailable, the application should allow the user to complete other tasks rather than crash completely.
- Fallback Mechanisms: Implement fallback mechanisms to handle known exceptions. For example, if a third-party service is temporarily down, the system can switch to a secondary service or queue requests until the primary service is back online.
- Don’t Catch Generic Exceptions: Avoid catching generic exceptions like
Exception
orThrowable
in your code. Catching specific exceptions allows for more precise error handling and prevents hiding other, unexpected errors.
6. Secure Coding Practices for Code Review and Testing
Regular code reviews and thorough testing are essential to ensure that your software is secure. These practices help identify vulnerabilities early in the development process, allowing you to fix them before they can be exploited.
Conducting Security-Focused Code Reviews
Code reviews play a vital role in the development process, ensuring quality and security. When performed with a focus on security, they can catch vulnerabilities that automated tools might miss.
- Peer Code Reviews: Have your peers review your code with security in mind. A fresh set of eyes can often catch mistakes that the original developer missed. Encourage your team to look for common security flaws, such as improper input validation, insufficient access controls, and the use of outdated libraries.
- Follow a Checklist: Use a secure coding checklist during code reviews to ensure that all potential security issues are considered. The OWASP (Open Web Application Security Project) Secure Coding Practices Checklist is a great resource to start with.
- Regular Reviews: Security-focused code reviews should be an ongoing part of the development process. Make sure that security checks are integrated into your version control system, so that every new commit is reviewed for potential security issues.
Using Static and Dynamic Analysis Tools
In addition to manual code reviews, automated tools can help identify vulnerabilities in your code.
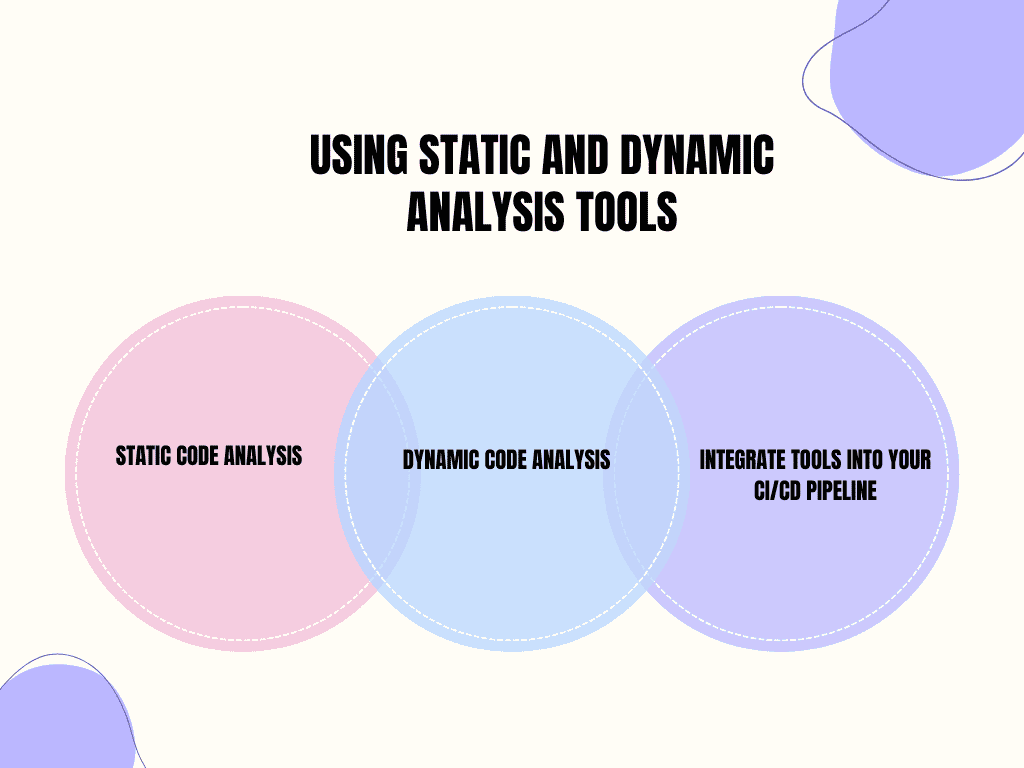
- Static Code Analysis: Static analysis tools examine your code without executing it, looking for common vulnerabilities such as buffer overflows, SQL injection risks, and XSS. Examples of popular static analysis tools include SonarQube, Veracode, and Fortify.
- Dynamic Code Analysis: Dynamic analysis tools test your application while it is running, simulating real-world attacks. These tools can identify issues that static analysis tools might miss, such as runtime vulnerabilities or configuration errors. Examples of dynamic analysis tools include Burp Suite and OWASP ZAP.
- Integrate Tools into Your CI/CD Pipeline: By integrating static and dynamic analysis tools into your continuous integration/continuous deployment (CI/CD) pipeline, you can automatically scan your code for vulnerabilities every time it is updated. This ensures that security testing is an ongoing process rather than an afterthought.
The Importance of Penetration Testing
Penetration testing, also known as ethical hacking, is the process of simulating real-world attacks on your software to identify security weaknesses.
- Hire a Penetration Tester: Penetration testers are security experts who specialize in finding vulnerabilities in software. Hiring a penetration tester can give you insights into how attackers might exploit your system and what you can do to protect against those attacks.
- Automated Penetration Testing Tools: While manual penetration testing is essential, automated tools like Metasploit can simulate common attacks and identify vulnerabilities that might otherwise go unnoticed.
- Regular Testing: Penetration testing should be performed regularly, especially after significant code changes or before major releases. This ensures that new features or fixes don’t introduce new vulnerabilities.
7. Secure Coding Practices for Third-Party Libraries
Most modern software projects rely on third-party libraries to speed up development. While these libraries can be extremely helpful, they also introduce potential security risks. Properly managing these dependencies is a critical part of secure coding.
Evaluating External Dependencies
Before incorporating third-party libraries into your project, it’s important to evaluate their security and reliability.
- Research the Library: Check the library’s track record for security. Does it have a history of vulnerabilities? How quickly are security issues addressed? Look for reviews, bug reports, and any signs that the library may not be actively maintained.
- Community Support: Libraries with a large, active community are generally more secure because bugs and vulnerabilities are found and fixed quickly. Look for libraries that have an active GitHub repository, frequent updates, and responsive maintainers.
- Minimal Dependencies: Avoid libraries that rely on many other dependencies, as each additional dependency introduces another potential point of failure. Choose libraries that are lightweight and self contained whenever possible.
Keeping Libraries Updated
Outdated libraries can be a major security risk. Many vulnerabilities are found and patched in newer versions of libraries, so keeping your dependencies up to date is crucial.
- Regularly Check for Updates: Use tools like Dependabot or Snyk to automatically check for updates to your third-party libraries. These tools can notify you when a new version is available, especially if it includes important security fixes.
- Test Updates in a Safe Environment: Before applying updates to third-party libraries in your production environment, test them in a staging or development environment to ensure that they don’t introduce compatibility issues or new bugs.
- Remove Unused Dependencies: Over time, you may accumulate libraries that are no longer in use. Regularly audit your project’s dependencies and remove any that are no longer necessary to reduce the attack surface of your application.
Minimizing Risks from External Components
While third-party libraries can save development time, they also come with security risks. You can minimize these risks by taking a few additional precautions.
- Isolate Third-Party Code: Where possible, run third-party libraries in a sandbox or isolated environment. This ensures that if the library is compromised, the damage is contained.
- Use Vendor-Supplied Security Updates: Many libraries have security patches that are released regularly. Make sure you’re subscribed to mailing lists or updates from the library maintainers so you can apply patches as soon as they become available.
- Limit Access: Give third-party libraries the minimum level of access they need to function. For example, if a library only needs to read data from a database, don’t grant it write access.
8. Secure Coding Practices for Secure Deployment
Even if your code is written securely, vulnerabilities can still be introduced during the deployment process. By following secure deployment practices, you can ensure that your application is protected from potential threats after it goes live.
Ensuring Secure Configuration
Misconfigured servers and environments are one of the most common causes of security breaches. It’s essential to configure your production environment securely before deploying your application.
- Disable Debugging and Error Reporting: Make sure that debugging tools and verbose error messages are disabled in the production environment. These features can provide attackers with valuable information about your system.
- Harden Your Server: Server hardening refers to the process of securing a server by reducing its attack surface. This includes disabling unnecessary services, closing unused ports, and setting strong firewall rules.
- Secure File Permissions: Ensure that files and directories in your production environment have the correct permissions. For example, sensitive configuration files should only be accessible to the application, not to users or other services.
Managing Updates and Patches
Security doesn’t stop at deployment. Once your application is live, it’s important to keep it up to date with the latest security patches.
- Apply Patches Promptly: Whenever security patches are released for your software stack (including the operating system, web server, database, and libraries), apply them as soon as possible. Delaying updates can leave your application vulnerable to known exploits.
- Automate Updates: Automating the update process using tools like Ansible or Chef can help ensure that patches are applied consistently and in a timely manner. Automation reduces the risk of human error and ensures that no important updates are missed.
- Monitor for Security Vulnerabilities: Stay informed about new vulnerabilities in the tools and libraries your application uses. Subscribe to security mailing lists, follow security blogs, and use vulnerability scanners to check your system for potential weaknesses.
Difference Between Static Code Analysis and Dynamic Code Analysis
Static Code Analysis | Dynamic Code Analysis |
---|
1. Analyzes the source code without executing it. | Tests the running application to find vulnerabilities. |
2. Identifies potential security issues early in the development process. | Detects runtime vulnerabilities during execution. |
3. Can be automated to scan code regularly. | Requires the application to be running, often in a test environment. |
4. Examples include tools like SonarQube and Checkmarx. | Examples include tools like OWASP ZAP and Burp Suite. |
5. Useful for enforcing coding standards and best practices. | Useful for simulating real-world attacks on the application. |
6. Does not require any test data to function. | Test data is required to execute the application properly. |
FAQs
1 What are secure coding practices?
Secure coding practices are a set of guidelines and techniques that developers use to write code that is resistant to security vulnerabilities. These practices help protect applications from common threats such as SQL injection, cross-site scripting (XSS), and unauthorized access.
2 Why is input validation important in secure coding?
Input validation is crucial because it ensures that the application processes only properly formatted and safe data. By validating and sanitizing user inputs, developers can prevent attacks that exploit untrusted data, such as SQL injection and XSS attacks.
3 How does encryption enhance software security?
Encryption protects sensitive data by converting it into a coded format that can only be read by authorized users with the correct decryption key. This prevents unauthorized access to data both in transit (when being sent over networks) and at rest (when stored).
4 What is the role of authentication and authorization in secure coding?
Authentication verifies the identity of users trying to access the application, while authorization determines what actions or resources those authenticated users can access. Implementing strong authentication and authorization mechanisms is vital for protecting sensitive data and preventing unauthorized access.
5 How can regular code reviews improve security?
Regular code reviews allow developers to examine code for potential vulnerabilities and security flaws before the software is deployed. This practice encourages collaboration and helps catch issues early in the development process, reducing the risk of exploitation in production environments.
9. Conclusion: Secure Coding Practices for the Future
As cyber threats continue to evolve, secure coding practices are becoming more important than ever. By following the guidelines outlined in this article, you can build software that is both functional and secure. These practices will help you protect your application from common vulnerabilities, reduce the risk of data breaches, and ensure that your software remains resilient against attacks. But secure coding is not a one-time task—it’s an ongoing commitment. As new threats emerge and technology evolves, it’s important to stay informed and continuously improve your security practices. This includes regularly reviewing your code, updating your dependencies, and incorporating new security tools and techniques into your development process.